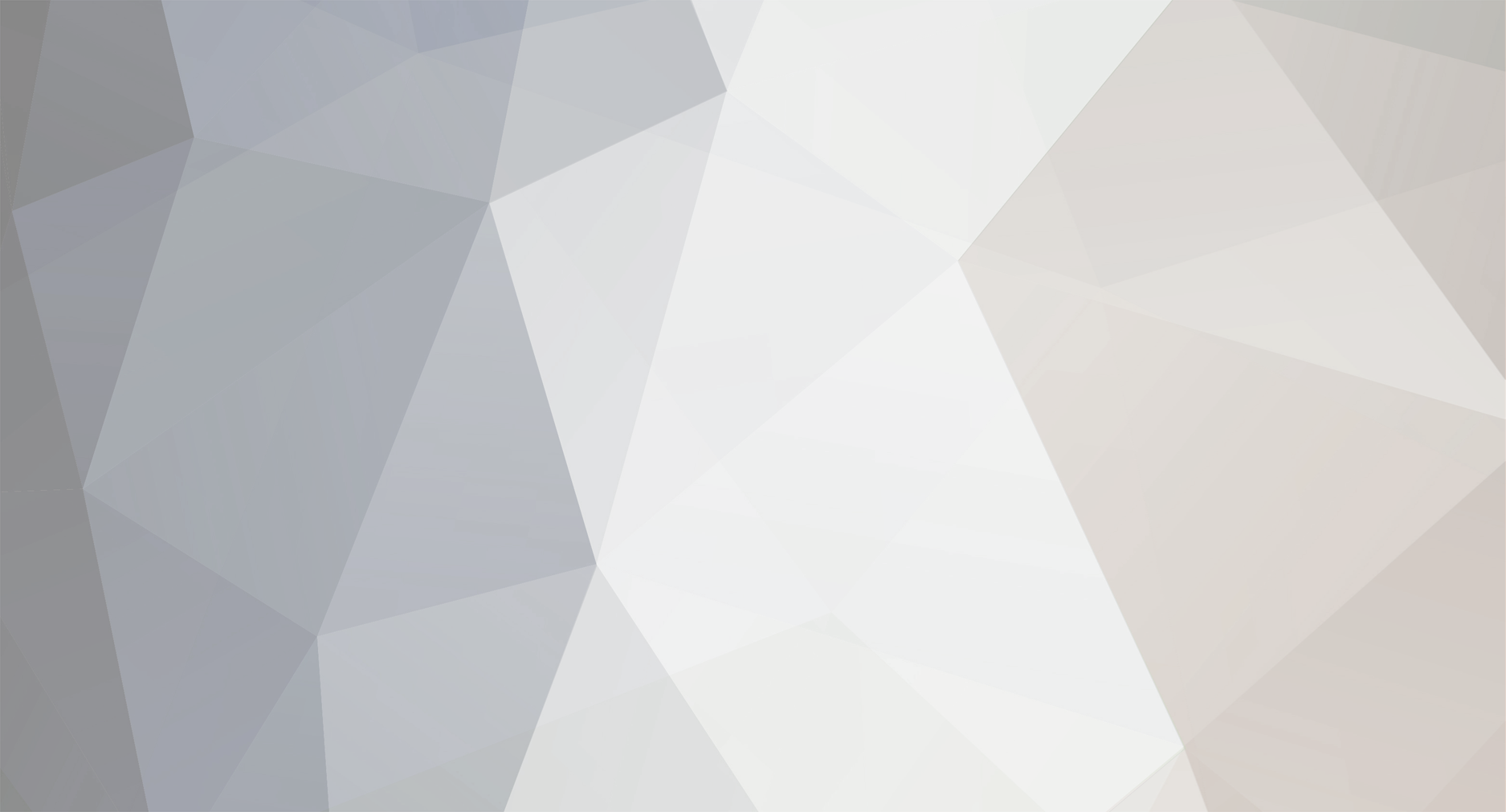
peterthepigeon
Members-
Posts
112 -
Joined
-
Last visited
Everything posted by peterthepigeon
-
Superweapon stuff in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
004712D3 8B56 08 MOV EDX,DWORD PTR DS:[ESI+8] ; Cases D,14 of switch 004713C0 004712D6 89F0 MOV EAX,ESI 004712D8 FF92 18010000 CALL DWORD PTR DS:[EDX+118] ; C&C95.00413D58 004712DE 8D7E 1E LEA EDI,DWORD PTR DS:[ESI+1E] 004712E1 8B15 D8DC5300 MOV EDX,DWORD PTR DS:[53DCD8] 004712E7 8D7424 30 LEA ESI,DWORD PTR SS:[ESP+30] 004712EB 894424 34 MOV DWORD PTR SS:[ESP+34],EAX 004712EF 895424 30 MOV DWORD PTR SS:[ESP+30],EDX 004712F3 A5 MOVS DWORD PTR ES:[EDI],DWORD PTR DS:[ESI] 004712F4 A5 MOVS DWORD PTR ES:[EDI],DWORD PTR DS:[ESI] 004712F5 81C4 98000000 ADD ESP,98 004712FB 5F POP EDI 004712FC 5E POP ESI 004712FD 5A POP EDX 004712FE 5B POP EBX 004712FF C3 RETN This code seems to "spawn" or maybe "draw" the A10s. It also controls the behavior. eg: 00413E92 C646 1D 02 MOV BYTE PTR DS:[ESI+1D],2 2 Tells the A10 to start it's bombing run. 00413DA9 C646 1D 01 MOV BYTE PTR DS:[ESI+1D],1 Setting the var to 1 A10 to circle around it's target, but doesn't drop bombs. I've not tested 0 yet. -
Superweapon stuff in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
Have you solved the airstrike logic for the number of planes? -
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
I've reversed games where they've done similar things to this, but the data was packed in HIWORD and LOWORD, or they would pack the data as DWORD and shift it out. I've amended it, but at the end of the day we can both say that it's stored as a DWORD but used as a BYTE. -
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
Quibbling over style is what OCD infested people do -
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
Right on the money. Now do you see why I did it as BYTE? I think at any rate, now that I think about it. Maybe they wanted to use bitshifting to pack data in the struct? -
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
004B0E52 |. 89C2 MOV EDX,EAX 004B0E54 |. C1FA 1F SAR EDX,1F 004B0E57 |. C1E2 08 SHL EDX,8 004B0E5A |. 1BC2 SBB EAX,EDX 004B0E5C |. C1F8 08 SAR EAX,8 SAR EAX, 8 -
Superweapon stuff in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
That call is the function which resets Ion Cannon, Airstrike and Nuke. -
C&C95 player production/building stuff
peterthepigeon replied to peterthepigeon's topic in The Tech Center
It's to show the maximum possible speed that can be obtained. As far as I've tested, I haven't determined if it affects the AI or not. I believe it would however. -
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
I reversed the struct that way because I felt it would make things clearer. The bitshifting done by the game to obtain data is a bit odd. As far as the naming convention goes, I find it odd that WW didn't think to more descriptively name their files. Also the range is just a BYTE which is bitshifted out of the DWORD. -
Let's start shall we? 0044C465 E8 523DFCFF CALL C&C95.004101BC This little pup resets the superweapon timers back when you use it. Haven't tested nuke yet. Within the body of the function, this little nugget: 004101D4 8828 MOV BYTE PTR DS:[EAX],CH Seems that when the superweapon is fully charged CH is 5. Also this: 0041029D 8D40 00 LEA EAX,DWORD PTR DS:[EAX] 004102A0 53 PUSH EBX 004102A1 51 PUSH ECX 004102A2 52 PUSH EDX 004102A3 8A10 MOV DL,BYTE PTR DS:[EAX] 004102A5 F6C2 01 TEST DL,1 004102A8 74 68 JE SHORT C&C95.00410312 004102AA F6C2 04 TEST DL,4 004102AD 74 09 JE SHORT C&C95.004102B8 004102AF B8 66000000 MOV EAX,66 004102B4 5A POP EDX 004102B5 59 POP ECX 004102B6 5B POP EBX 004102B7 C3 RETN I'll update this post when I reverse more. Maybe a full week down the road. Ion Cannon damage and so on I was working on but lost the data.
-
While I was munging around in C&C95 using my old trusty tools, T-Search, IDA, and Olly, I found the algorithim where the game determines how long it takes a structure/unit to build. I have reason to believe the superweapons may have similar logic to this: BYTE bTicksBetweenBuildCall = ( unsigned short )( Cost / 0x6C ); That little pup calculates how many ticks must pass before the game calls the continue build algorithm again. I may be wrong however in the exact wording but that's what I've been able to determine. Now the actual total ticks before it begins the sequence all over again in the build call is 0x6C. I've determined that you can at most increase the base speed by 4. Beyond that it doesn't work. Now onto the programmer stuff again. 0043C000 |. 8846 02 MOV BYTE PTR DS:[ESI+2],AL 0043C003 |. 80E2 FD AND DL,0FD 0043C006 |. 8846 03 MOV BYTE PTR DS:[ESI+3],AL AL is the calculated bTicksBetweenBuildCall. 0043BDE1 8A4B 02 MOV CL,BYTE PTR DS:[EBX+2] 0043BDE4 FEC9 DEC CL 0043BDE6 |. 884B 02 MOV BYTE PTR DS:[EBX+2],CL Obviously decrements the stored bTicksBetweenBuildCall until it hits 0. Then the whole thing starts again. 0043BDF2 8A43 03 MOV AL,BYTE PTR DS:[EBX+3] 0043BDF5 42 INC EDX 0043BDF6 8843 02 MOV BYTE PTR DS:[EBX+2],AL This pup resets the bTicksBetweenBuildCall back to normal and increments the bBuildTicks. Now to explain what I meant earlier, bBuildTicks can only be increased by a max of 4 including the increment. If you also zero the bTicksBetweenBuildCall and add 4 to bBuildTicks, you will achieve maximum producton speed in the game. 0043BDE1 32C9 XOR CL,CL 0043BDE3 90 NOP 0043BDE4 90 NOP 0043BDE5 90 NOP 0043BDE6 |. 884B 02 MOV BYTE PTR DS:[EBX+2],CL 0043BDE9 |.^0F85 55FFFFFF JNZ C&C95.0043BD44 0043BDEF |. 66:8B13 MOV DX,WORD PTR DS:[EBX] 0043BDF2 66:83C2 04 ADD DX,4 0043BDF6 8843 02 MOV BYTE PTR DS:[EBX+2],AL
-
Weapons Research in Tiberian Dawn
peterthepigeon replied to peterthepigeon's topic in The Tech Center
Continuing on, there are a max of 18 projectiles. 004B62FD |. 8B1495 BC295000 MOV EDX,DWORD PTR DS:[EDX*4+5029BC] 5029BC is the projectile base. Projectile 00 - 50cal Projectile 01 - 50cal Projectile 02 - 120mm Projectile 03 - 120mm Projectile 04 - DRAGON Projectile 05 - DRAGON Projectile 06 - MISSILE Projectile 07 - DRAGON Projectile 08 - FLAME Projectile 09 - FLAME Projectile 10 - BOMBLET Projectile 11 - BOMB Projectile 12 - Laser Projectile 13 - ATOMICUP Projectile 14 - ATOMICDN Projectile 15 - MISSILE Projectile 16 - 50cal Projectile 17 - GORE Projectile 18 - CHEW -
00438719 8B40 48 MOV EAX,DWORD PTR DS:[EAX+48] 0043871C F640 05 02 TEST BYTE PTR DS:[EAX+5],2 00438720 74 07 JE SHORT C&C952.00438729 00438722 B8 19000000 MOV EAX,19 00438727 5A POP EDX 00438728 C3 RETN 00438729 B8 C4020000 MOV EAX,2C4 0043872E 5A POP EDX 0043872F C3 RETN TEST BYTE PTR DS:[EAX+5],2 This tells us if this is the computer player or the human player. Now to answer what the non RE programmers are asking. What is going on? The answer is trivial, the computer player and human player's harvesters both carry 28 bails of tiberium. This is obvious, but what isn't so obvious is that the computer's bails are worth an astounding 708 credits per bail vs the 25 credits the human player gets. That's really it, to be honest.
-
A repost of what I posted on dune2k forum. From start to finish? Maybe 4 hours of on and off work to put it all together. Nyerguds weapons.ini had the indices which made testing easier. So let's get to work. Where is the weapons' data stored? You can find it in the .data section of c&c, specifically in C&C95, at address 0x5034D8. 0044114A |. 8A84C1 D834500>MOV AL,BYTE PTR DS:[ECX+EAX*8+5034D8] To walk the entries, it's important to remember there are a total of 24(?) weapons. #define MAX_WEAPONS 24 typedef struct { BYTE m_bProjectile; BYTE m_bDamage; BYTE m_bRateOfFire; DWORD m_dwRange; // BYTE m_bRange * 0x100, bRange = m_bRange >> 8; BYTE m_bReportSound; }Weapon_t; Weapon_t* pWeaponBase = ( Weapon_t* )0x5034D8; for ( iCounter = 0; iCounter < MAX_WEAPONS; iCounter++ ) { SetupWeapon ( &pWeaponBase [ iCounter ], iCounter ); } Keep in mind that Rate of Fire has 3 added to it by the game. To truly have it set to 0 you either: C&C95 location: 004B1766 004B1766 |. 83C0 03 ADD EAX,3 1) Byte patch 1a) Byte patch immediate to 0 2) Set Rate of Fire to FD to cause overflow. This works because game only uses bytes for weapon data as documented above.